Steps To Implement Stack In Java | Simple Data Structure Introduction
Agenda :
1) What is Stack
2) What are the methods or operations performed in stack
3) What is the Time complexity of stack
4) How can we implement a stack
5) Simple example with output
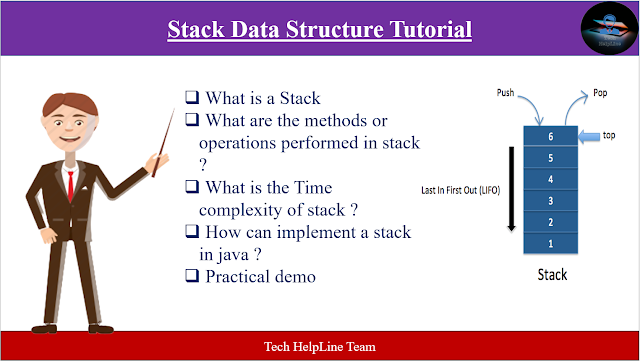
1) What is Stack :
Thanks,
The Tech HelpLine Team :)
Email ID : jobsmails18@gmail.com
Facebook Channel : https://www.facebook.com/pg/Tech-HelpLine-107359517579302
YouTube Channel : https://www.youtube.com/channel/UCFCIUqpwCMl4i7Lqtovz_cQ
1) What is Stack
2) What are the methods or operations performed in stack
3) What is the Time complexity of stack
4) How can we implement a stack
5) Simple example with output
1) What is Stack :
- Stack is a linear data structure, which follows the sequential order.
- It followed the LIFO(Last In First Out) or FILO(First In Last Out) insertion order.
2) What are the methods or operations performed in stack :
- Basically there are five type of operations are available in stack and these are below,
- Push: This method will add the elements in stack if stack is not full, if it's full then it will show an overflow condition.
- Pop: This method will remove the elements from the stack. And elements are get removed by the reversed order in which they are pushed. If the stack is empty, then it will show an underflow condition.
- Peek or Top: This method will returns top element of stack.
- isEmpty: This method will returns true if stack is empty, else false.
- isFull: This method will returns true if stack is full, else false.
3) What is the Time complexity of stack
- push(), pop(), isEmpty(), isFull() and peek() all take O(1) time. We do not run any loop in any of these operations.
4) How can we implement a stack
- There are two ways to implement a stack:
- Using array
- Using linked list
5) Simple example using array
Input :
package com.techhelpline.stack;
public class StackImplementation {
int stackSize;
int arr[];
int topElement;
public StackImplementation(int sizeP) {
this.stackSize = sizeP;
this.arr = new int[stackSize];
this.topElement = -1;
}
public void push(int pushNumber) {
if (!isFull()) {
topElement++;
arr[topElement] = pushNumber;
System.out.println("Element " + pushNumber + " is pushed in stack!!!");
} else {
System.out.println("Stack is already fulled!!");
}
}
public int pop() {
if (!isEmpty()) {
int popValue = topElement;
topElement--;
System.out.println("Poped value is : " + arr[popValue]);
return arr[popValue];
} else {
System.out.println("Stack is empty!!!!");
return -1;
}
}
public boolean isEmpty() {
return (topElement == -1);
}
public boolean isFull() {
return (stackSize - 1 == topElement);
}
public int peek() {
if (!this.isEmpty()) {
return arr[topElement];
} else {
System.out.println("Stack is empty!!!");
return -1;
}
}
public static void main(String[] args) {
StackImplementation implementation = new StackImplementation(10);
implementation.pop();
System.out.println("------------------");
implementation.push(10);
implementation.push(20);
implementation.push(30);
implementation.push(40);
System.out.println("------------------");
int peekValue = implementation.peek();
System.out.println("Peek Element Value: " + peekValue);
System.out.println("------------------");
implementation.pop();
implementation.pop();
implementation.pop();
implementation.pop();
System.out.println("------------------");
System.out.println("Stack is Empty? :" + implementation.isEmpty());
System.out.println("------------------");
System.out.println("Stack is Full? :" + implementation.isFull());
}
}
Output :
Stack is empty!!!!
------------------
Element 10 is pushed in stack!!!
Element 20 is pushed in stack!!!
Element 30 is pushed in stack!!!
Element 40 is pushed in stack!!!
------------------
Peek Element Value: 40
------------------
Poped value is : 40
Poped value is : 30
Poped value is : 20
Poped value is : 10
------------------
Stack is Empty? :true
------------------
Stack is Full? :false
public class StackImplementation {
int stackSize;
int arr[];
int topElement;
public StackImplementation(int sizeP) {
this.stackSize = sizeP;
this.arr = new int[stackSize];
this.topElement = -1;
}
public void push(int pushNumber) {
if (!isFull()) {
topElement++;
arr[topElement] = pushNumber;
System.out.println("Element " + pushNumber + " is pushed in stack!!!");
} else {
System.out.println("Stack is already fulled!!");
}
}
public int pop() {
if (!isEmpty()) {
int popValue = topElement;
topElement--;
System.out.println("Poped value is : " + arr[popValue]);
return arr[popValue];
} else {
System.out.println("Stack is empty!!!!");
return -1;
}
}
public boolean isEmpty() {
return (topElement == -1);
}
public boolean isFull() {
return (stackSize - 1 == topElement);
}
public int peek() {
if (!this.isEmpty()) {
return arr[topElement];
} else {
System.out.println("Stack is empty!!!");
return -1;
}
}
public static void main(String[] args) {
StackImplementation implementation = new StackImplementation(10);
implementation.pop();
System.out.println("------------------");
implementation.push(10);
implementation.push(20);
implementation.push(30);
implementation.push(40);
System.out.println("------------------");
int peekValue = implementation.peek();
System.out.println("Peek Element Value: " + peekValue);
System.out.println("------------------");
implementation.pop();
implementation.pop();
implementation.pop();
implementation.pop();
System.out.println("------------------");
System.out.println("Stack is Empty? :" + implementation.isEmpty());
System.out.println("------------------");
System.out.println("Stack is Full? :" + implementation.isFull());
}
}
Output :
Stack is empty!!!!
------------------
Element 10 is pushed in stack!!!
Element 20 is pushed in stack!!!
Element 30 is pushed in stack!!!
Element 40 is pushed in stack!!!
------------------
Peek Element Value: 40
------------------
Poped value is : 40
Poped value is : 30
Poped value is : 20
Poped value is : 10
------------------
Stack is Empty? :true
------------------
Stack is Full? :false
-----------------------------END----------------------------------
The Tech HelpLine Team :)
Email ID : jobsmails18@gmail.com
Facebook Channel : https://www.facebook.com/pg/Tech-HelpLine-107359517579302
YouTube Channel : https://www.youtube.com/channel/UCFCIUqpwCMl4i7Lqtovz_cQ
Comments
Post a Comment